MisterTootor M.S., B.S., A.S., A.S.B
Basic Program
​
<!DOCTYPE html>
<html>
<body>
<p>This is a sentence.</p>
<p>This is a sentence.</p>
<p>This is a sentence.</p>
</body>
</html>
​
Output
​
This is a sentence.
This is a sentence.
This is a sentence.
Change Background Color
​
<!DOCTYPE html>
<html>
<body style="background-color:powderblue;">
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
Output
​
​
This is a heading
This is a paragraph.
Bold Formatting
​
<!DOCTYPE html>
<html>
<body>
<p>This text is normal.</p>
<p><b>This text is bold.</b></p>
</body>
</html>
​
Output
​
This text is normal.
This text is bold.
Using “del” to delete
<!DOCTYPE html>
<html>
<body>
<p>My favorite color is <del>blue</del> red.</p>
</body>
</html>
Output
​
​
​
My favorite color is blue red.
How to use comments for debugging
<!DOCTYPE html>
<html>
<body>
<p>This is a paragraph.</p>
<!--
<p>Look at this cool image:</p>
<img border="0" src="pic_trulli.jpg" alt="Trulli">
-->
<p>This is a paragraph too.</p>
</body>
</html>
Output
​
This is a paragraph.
This is a paragraph too.
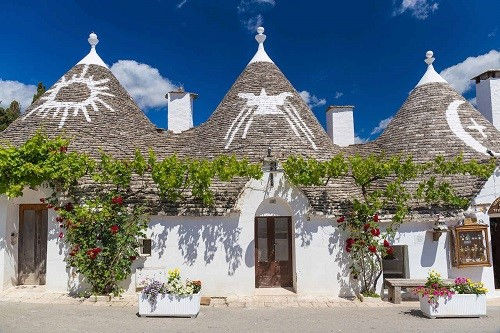
Inserting an Image
​
<!DOCTYPE html>
<html>
<body>
<h2>Italian Trulli</h2>
<img src="pic_trulli.jpg" alt="Italian Trullti" style="width:100%">
</body>
</html>image
Output
​
​
Italian Trulli

Inserting Animated images
<!DOCTYPE html>
<html>
<body>
<h2>Animated Images</h2>
<p>HTML allows moving images:</p>
<img src="programming.gif" alt="Computer man" style="width:48px;height:48px;">
</body>
</html>
Output
Animated Images
HTML allows moving images:
​
​
​
Clickable Areas
​
<!DOCTYPE html>
<html>
<body>
<h2>Image Maps</h2>
<p>Click on the sun or on one of the planets to watch it closer:</p>
<img src="planets.gif" alt="Planets" usemap="#planetmap" style="width:145px;height:126px;">
<map name="planetmap">
<area shape="rect" coords="0,0,82,126" alt="Sun" href="sun.htm">
<area shape="circle" coords="90,58,3" alt="Mercury" href="mercur.htm">
<area shape="circle" coords="124,58,8" alt="Venus" href="venus.htm">
</map>
</body>
</html>
​
Output
Image Maps
Click on the sun or on one of the planets to watch it closer:
​
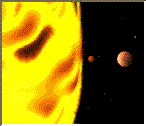
Table with borders
​
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
}
</style>
</head>
<body>
<h2>Table With Border</h2>
<p>Use the CSS border property to add a border to the table.</p>
<table style="width:100%">
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Age</th>
</tr>
<tr>
<td>Joe</td>
<td>blow</td>
<td>20</td>
</tr>
<tr>
<td>Harry</td>
<td>Harold</td>
<td>100</td>
</tr>
<tr>
<td>Joe</td>
<td>Doaks</td>
<td>110</td>
</tr>
</table>
</body>
</html>
Output
Table With Border
Use the CSS border property to add a border to the table.
​
​
Firstname Lastname Age
Joe blow 20
Harry Harold 100
Joe Doaks 110
Adding a YouTube Video with object
​
<?xml version="1.0" ?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" lang="en" xml:lang="en">
<head>
<title>Adding a YouTube Video</title>
</head>
<body>
<h1>Embedding a YouTube Video</h1>
<object width="1280" height="720"><param name="movie" value="http://www.youtube.com/watch?v=bnkI8tBNrPY"></param><param name="allowFullScreen" value="true"></param><param name="allowscriptaccess" value="always"></param><embed src="http://www.youtube.com/watch?v=bnkI8tBNrPY" type="application/x-shockwave-flash" allowscriptaccess="always" allowfullscreen="true" width="1280" height="720"></embed></object>
</body>
</html>
Adding an MP3 player using Flash
​
<?xml version="1.0" ?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" lang="en" xml:lang="en">
<head>
<title>Adding an MP3 player using Flash</title>
</head>
<body>
<h2>Flash MP3 Player Using object element</h2>
<object type="application/x-shockwave-flash" width="600" height="240"
data="flash/xspf_player.swf?playlist_url=flash/playlist.xspf">
<param name="movie" value="flash/xspf_player.swf?playlist_url=flash/playlist.xspf" />
</object>
</body>
</html>
​
Adding an MP3 without specifying player
​
<?xml version="1.0" ?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" lang="en" xml:lang="en">
<head>
<title>Adding an MP3 without specifying player</title>
</head>
<body>
<object width="400" height="80" type="audio/mpeg">
<param name="src" value="Clementine.mp3" />
<param name="autoplay" value="true" />
<param name="autostart" value="1" />
<embed src="Clementine.mp3" width="400" height="80" ></embed>
</object>
</body>
</html>
Building a Basic Form
​
<!DOCTYPE html>
<title>My Example</title>
<form method="get" action="/html/codes/html_form_handler.cfm">
<p>
<label>Name
<input type="text" name="customer_name" required>
</label>
</p>
<p>
<label>Phone
<input type="tel" name="phone_number">
</label>
</p>
<p>
<label>Email
<input type="email" name="email_address">
</label>
</p>
<fieldset>
<legend>Which Uber do you require?</legend>
<p><label> <input type="radio" name="taxi" required value="car"> Car </label></p>
<p><label> <input type="radio" name="taxi" required value="van"> Van </label></p>
<p><label> <input type="radio" name="taxi" required value="SUV"> SUV </label></p>
</fieldset>
<fieldset>
<legend>Extras</legend>
<p><label> <input type="checkbox" name="extras" value="baby"> Car Seat </label></p>
<p><label> <input type="checkbox" name="extras" value="wheelchair"> Wheelchair Access </label></p>
<p><label> <input type="checkbox" name="extras" value="stroller"> Stroller </label></p>
</fieldset>
<p>
<label>Pickup Date/Time
<input type="datetime-local" name="pickup_time" required>
</label>
</p>
<p>
<label>Pickup Place
<select id="pickup_place" name="pickup_place">
<option value="" selected="selected">Select One</option>
<option value="office" >Office</option>
<option value="residence" >Residence</option>
<option value="store" >Store</option>
</select>
</label>
</p>
<p>
<label>Dropoff Place
<input type="text" name="dropoff_place" required list="destinations">
</label>
<datalist id="destinations">
<option value="office">
<option value="residence">
<option value="store">
</datalist>
</p>
<p>
<label>Special Instructions
<textarea name="comments" maxlength="500"></textarea>
</label>
</p>
<p><button>Submit Booking</button></p>
</form>
<hr>
<p>More info: <a href="/html/tags/html_form_tag.cfm"><code>form</code></a>, <a href="/html/tutorial/html_forms.cfm">HTML Form Tutorial</a>, <a href="/html/codes/html_form_code.cfm">HTML Form Code</a>, <a href="/html/codes/html_form_to_email.cfm">Form to Email</a>.</p>
​
​
​